Oracle Technical Interview Questions part 3
- Oracle Technical Interview Questions part 3
- Q1) What are the different types of indexes in Oracle, and when would you use each type?
- 1. B-tree Indexes
- 2. Bitmap Indexes
- 3. Clustered Indexes
- 4. Function-Based Indexes
- 5. Reverse Key Indexes
- 6. Composite Indexes
- 7. Domain Indexes
- 8. Global and Local Partitioned Indexes
- Q2)How do you implement a recursive query in Oracle SQL? Provide an example.(Oracle Technical Interview Questions part 3)
- Example Table Structure
- Recursive Query to Find All Employees Reporting to a Manager
- Explanation
- Q3) What is the purpose of the MERGE statement, and how does it work?(Oracle Technical Interview Questions part 3)
- Purpose of the MERGE Statement
- How It Works
- Example
- MERGE Statement
- Result After Execution
- Benefits
- Q4) Explain the difference between correlated and non-correlated subqueries. Provide examples.(Oracle Technical Interview Questions part 3)
- Correlated Subqueries
- Non-Correlated Subqueries
- Key Differences
- Q5) What are analytical functions in Oracle? Give an example using RANK() or DENSE_RANK()
- What Are Analytical Functions in Oracle?
- Example Using RANK() and DENSE_RANK()
- Key Differences:
- Explanation:
Oracle Technical Interview Questions part 3
Q1) What are the different types of indexes in Oracle, and when would you use each type?
A:
Oracle offers several types of indexes, each suited for different use cases to optimize query performance. Here’s a rundown of the main ones:
1. B-tree Indexes
Use case: General-purpose indexing. Details: These are the default index type in Oracle. They provide fast access to rows for equality and range queries. Suitable for most indexing needs.
2. Bitmap Indexes
Use case: Low-cardinality columns (columns with few distinct values). Details: Ideal for columns with a limited number of distinct values, such as gender or status flags. Bitmap indexes are efficient for complex queries involving multiple conditions (e.g., OLAP).
3. Clustered Indexes
Use case: Tables clustered together on disk. Details: These indexes are used with clustered tables. They can improve performance for joins between tables that are often queried together.
4. Function-Based Indexes
Use case: Columns involving expressions or functions. Details: Allows indexing on expressions or functions applied to columns, rather than just the column values. Useful when queries involve calculations or transformations.
5. Reverse Key Indexes
Use case: Columns with monotonically increasing values (e.g., sequence numbers). Details: Reverses the bytes of the indexed column. This helps distribute index entries more evenly across the index blocks, reducing contention and improving performance.
6. Composite Indexes
Use case: Multiple columns indexing. Details: Combines multiple columns into a single index. Useful for queries that filter or sort based on multiple columns.
7. Domain Indexes
Use case: Specialized applications (e.g., spatial, text). Details: These are custom indexes created using Oracle’s Data Cartridge framework. Useful for specialized data types like spatial or text.
8. Global and Local Partitioned Indexes
Use case: Large partitioned tables. Details: Indexes that align with table partitions. Global partitioned indexes can span multiple partitions, while local partitioned indexes align with individual table partitions. Useful for improving performance and manageability of partitioned tables. (Oracle Technical Interview Questions part 3)
Q2)How do you implement a recursive query in Oracle SQL? Provide an example.(Oracle Technical Interview Questions part 3)
A:
In Oracle SQL, you can implement a recursive query using a Common Table Expression (CTE) with the WITH
clause. This type of query is particularly useful for hierarchical or tree-structured data, such as organizational charts, bill of materials, or directory structures.
Here’s a step-by-step example of a recursive query to find all employees in an organization reporting to a specific manager:
Example Table Structure
Assume we have a table called EMPLOYEES
with the following structure:
EMPLOYEE_ID | EMPLOYEE_NAME | MANAGER_ID |
---|---|---|
1 | Alice | null |
2 | Bob | 1 |
3 | Carol | 1 |
4 | Dave | 2 |
5 | Eve | 2 |
Recursive Query to Find All Employees Reporting to a Manager
— Anchor member: Start with the root manager (e.g., employee with ID 1)
SELECT
EMPLOYEE_ID,
EMPLOYEE_NAME,
MANAGER_ID,
LEVEL AS HIERARCHY_LEVEL
FROM
EMPLOYEES
WHERE
EMPLOYEE_ID = 1
UNION ALL
— Recursive member: Join employees with their managers
SELECT
e.EMPLOYEE_ID,
e.EMPLOYEE_NAME,
e.MANAGER_ID,
eh.HIERARCHY_LEVEL + 1
FROM
EMPLOYEES e
JOIN EmployeeHierarchy eh ON e.MANAGER_ID = eh.EMPLOYEE_ID
)
SELECT
EMPLOYEE_ID,
EMPLOYEE_NAME,
MANAGER_ID,
HIERARCHY_LEVEL
FROM
EmployeeHierarchy
ORDER BY
HIERARCHY_LEVEL;
Explanation
- Anchor Member: The initial part of the CTE selects the root manager (in this case, Alice with
EMPLOYEE_ID = 1
). - Recursive Member: The recursive part of the CTE joins the
EMPLOYEES
table with the result of the previous level, effectively traversing the hierarchy. - Final SELECT Statement: The main query selects from the CTE and orders the results by the hierarchy level.
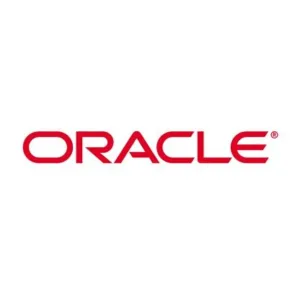
Oracle interview questions. Pic credit: Pinterest
Q3) What is the purpose of the MERGE statement, and how does it work?(Oracle Technical Interview Questions part 3)
A:
MERGE
statement in Oracle is used to perform an upsert operation—a combination of update and insert—in a single SQL statement. It is particularly useful when you need to either update existing rows in a target table or insert new rows when they do not exist.Result After Execution
Updated employees
Table:
employee_id | name | salary |
---|---|---|
101 | John | 55000 |
102 | Alice | 60000 |
103 | Bob | 45000 |
Benefits
- Simplicity: Single statement for both updates and inserts.
- Performance: Reduces the overhead of multiple operations and table scans.
- Clarity: Code is more readable and easier to maintain.